π Mastering SQL Queries: Understanding SELECT, WHERE, and ORDER BY
Mastering SQL Queries: SQL queries are the foundation of database management, allowing you to retrieve, filter, and organize data efficiently. Three essential SQL commands that every beginner should master are:
β
SELECT β Retrieve data from a table
β
WHERE β Filter data based on conditions
β
ORDER BY β Sort query results
By the end of this guide, you’ll be able to write SQL queries to fetch and organize data effectively. π
π 1. The SQL SELECT Statement
The SELECT
statement is used to retrieve data from a table.
Basic SELECT Query Example
SELECT * FROM Customers;
β
The *
symbol means select all columns from the Customers table.
β
This query returns every record stored in the database.
Selecting Specific Columns
Instead of selecting all columns, you can specify the ones you need.
SELECT Name, Email FROM Customers;
β This query retrieves only the Name and Email columns.
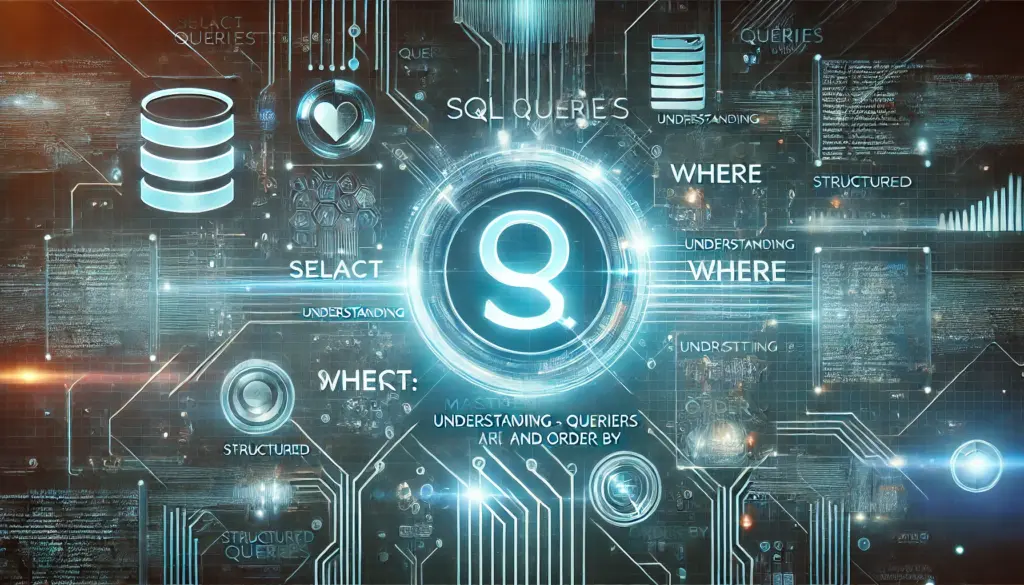
π 2. Filtering Data with the WHERE Clause
The WHERE
clause is used to filter records based on conditions.
Example Query: Get Customers from New York
SELECT * FROM Customers WHERE City = 'New York';
β This query returns only customers from New York
Using Comparison Operators in WHERE
Operator | Description | Example |
---|---|---|
= | Equal to | WHERE Age = 30 |
> | Greater than | WHERE Age > 25 |
< | Less than | WHERE Age < 40 |
!= or <> | Not equal to | WHERE City != 'Chicago' |
LIKE | Pattern matching | WHERE Name LIKE 'A%' |
IN | Match multiple values | WHERE City IN ('New York', 'Chicago') |
Other SQL Operators:
Operator | Description | Example |
---|---|---|
BETWEEN | Within a range (inclusive) | SELECT * FROM sales WHERE amount BETWEEN 500 AND 1000; |
IN | Matches any value in a list | SELECT * FROM customers WHERE city IN ('New York', 'Los Angeles', 'Chicago'); |
NOT IN | Excludes values in a list | SELECT * FROM employees WHERE role NOT IN ('Manager', 'Director'); |
LIKE | Pattern matching with wildcards | SELECT * FROM users WHERE name LIKE 'J%'; |
NOT LIKE | Excludes pattern matches | SELECT * FROM emails WHERE subject NOT LIKE '%offer%'; |
IS NULL | Checks for NULL values | SELECT * FROM orders WHERE delivery_date IS NULL; |
IS NOT NULL | Checks for non-NULL values | SELECT * FROM orders WHERE delivery_date IS NOT NULL; |
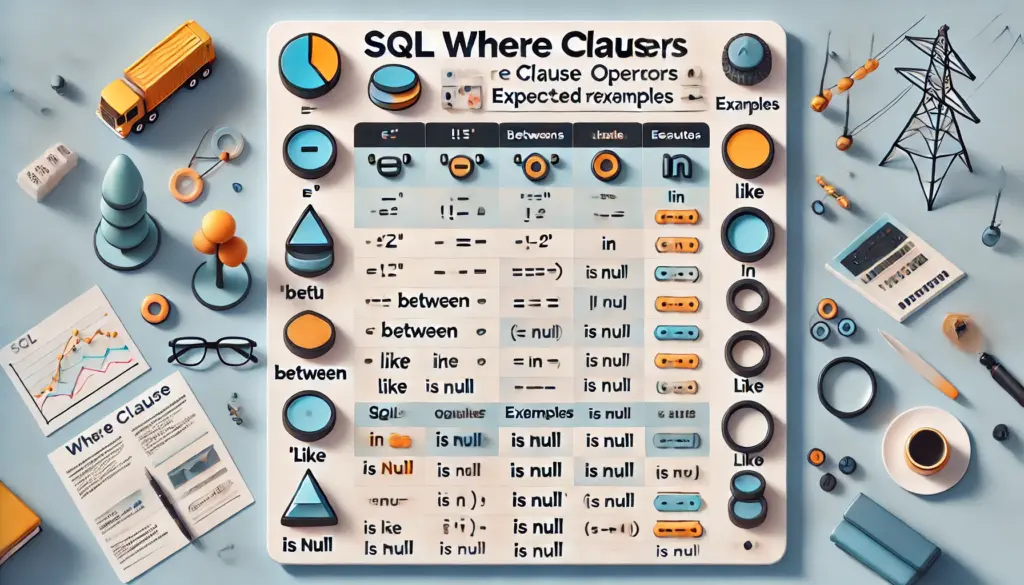
π 3. Sorting Data with ORDER BY
The ORDER BY
clause is used to sort query results in ascending or descending order.
Example Query: Sort Customers Alphabetically
SELECT * FROM Customers ORDER BY Name ASC;
β
ASC
= Ascending order (A β Z)
β
DESC
= Descending order (Z β A)
Sorting by Multiple Columns
SELECT * FROM Customers ORDER BY City ASC, Name DESC;
β Sorts customers by city first, then by name in descending order.
π 4. Combining SELECT, WHERE, and ORDER BY
SQL allows combining multiple clauses to create complex queries.
Example Query: Get Customers from New York & Sort by Name
SELECT Name, Email FROM Customers WHERE City = 'New York' ORDER BY Name ASC;
β
Filters customers from New York
β
Sorts them alphabetically
π 5. Common Mistakes to Avoid in SQL Queries
π« Forgetting to use quotes for text values:
β Incorrect
SELECT * FROM Customers WHERE City = New York;
β Fix:
SELECT * FROM Customers WHERE City = 'New York';
π« Using WHERE with aggregate functions (Use HAVING instead):
β Incorrect
SELECT COUNT(*) FROM Customers WHERE COUNT(*) > 10;
β Fix:
SELECT COUNT(*) FROM Customers GROUP BY City HAVING COUNT(*) > 10;
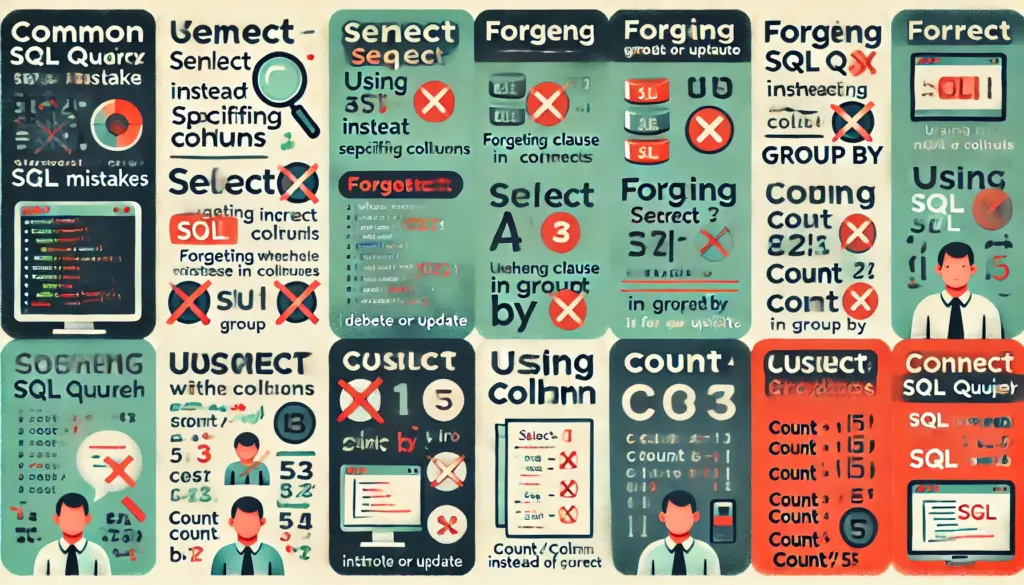
π Final Thoughts: Why Master These SQL Queries?
Mastering SELECT, WHERE, and ORDER BY is essential for:
β Data retrieval and reporting π
β Managing customer information in databases π’
β Analyzing business data for insights π
Next up in the series: “Understanding SQL Joins: INNER, LEFT, RIGHT, and FULL Joins Explained” β Stay tuned! π