π Understanding SQL Joins: INNER, LEFT, RIGHT, and FULL Joins Explained
SQL Joins allow you to combine data from multiple tables based on a related column. Mastering Joins is essential for working with relational databases.
In this guide, you’ll learn:
β What SQL Joins are and why they are important
β The difference between INNER, LEFT, RIGHT, and FULL Joins
β Practical examples with step-by-step explanations
By the end of this post, youβll be able to efficiently join multiple tables in SQL. π
π 1. What Are SQL Joins?
In relational databases, data is stored in separate tables to reduce redundancy. SQL Joins allow us to retrieve related data from multiple tables in a single query.
Example: A company has two tables:
Customers Table
CustomerID | Name | City |
---|---|---|
1 | Alice | New York |
2 | Bob | Chicago |
3 | Charlie | Miami |
Orders Table
OrderID | CustomerID | Product |
---|---|---|
101 | 1 | Laptop |
102 | 2 | Phone |
103 | 4 | Tablet |
β
The CustomerID
column is the common link between these tables.
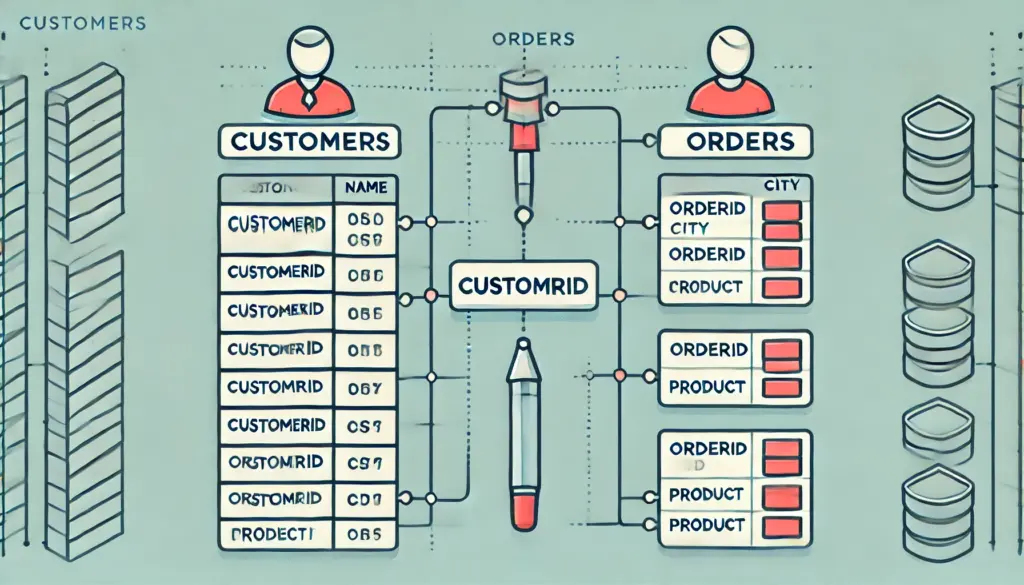
π 2. INNER JOIN β Returning Matching Records
The INNER JOIN returns only records with matching values in both tables.
Example Query: Get Customers Who Placed Orders
SELECT Customers.Name, Orders.Product
FROM Customers
INNER JOIN Orders ON Customers.CustomerID = Orders.CustomerID;
INNER JOIN Result
Name | Product |
---|---|
Alice | Laptop |
Bob | Phone |
β Only customers with orders appear in the results.
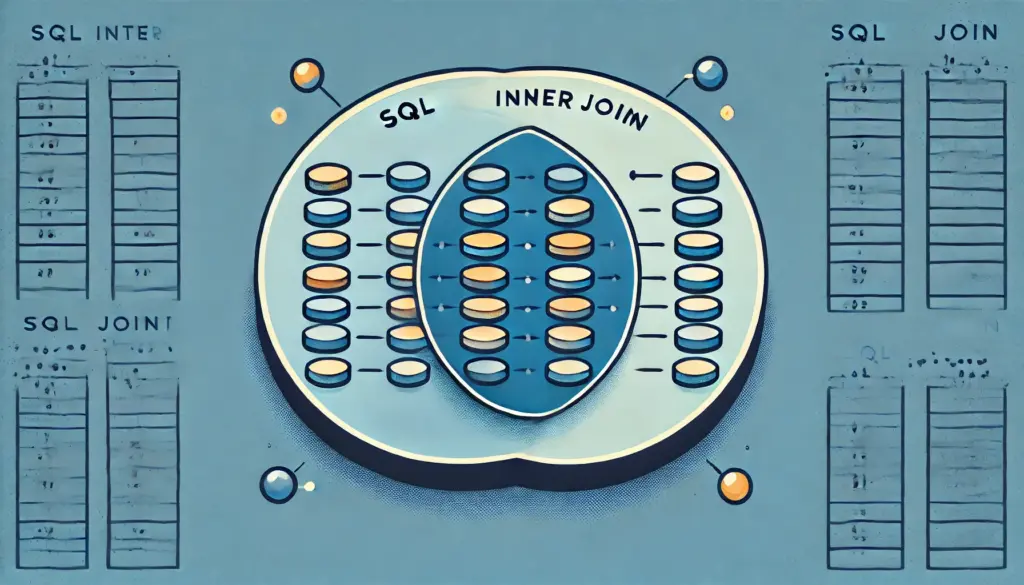
π 3. LEFT JOIN β Keeping All Records from the Left Table
The LEFT JOIN returns all records from the left table (Customers) and matching records from the right table (Orders). If no match is found, NULL is returned.
Example Query: Get All Customers and Their Orders
SELECT Customers.Name, Orders.Product
FROM Customers
LEFT JOIN Orders ON Customers.CustomerID = Orders.CustomerID;
LEFT JOIN Result
Name | Product |
---|---|
Alice | Laptop |
Bob | Phone |
Charlie | NULL |
β Charlie appears even though he has no order.
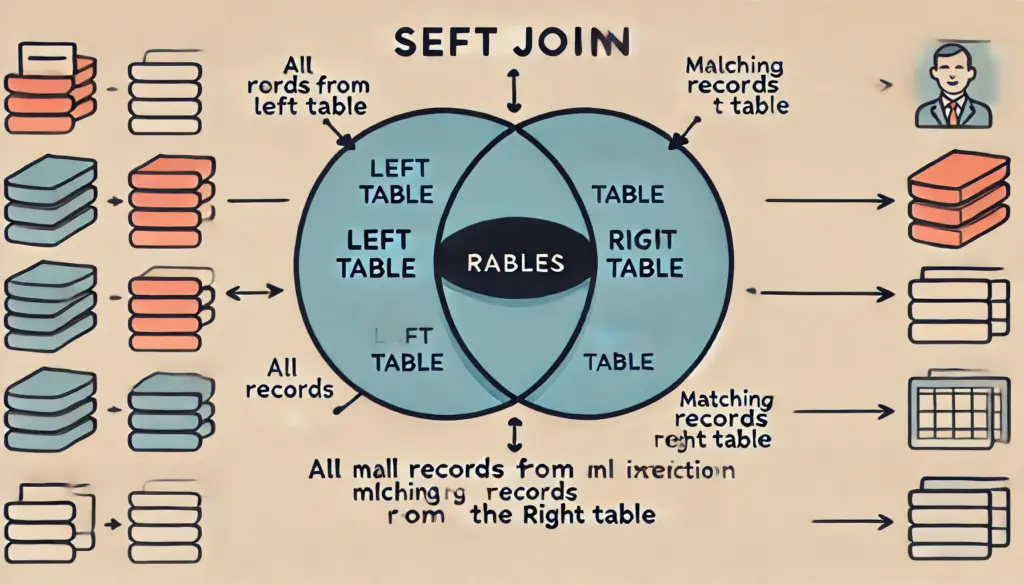
π 4. RIGHT JOIN β Keeping All Records from the Right Table
The RIGHT JOIN is the opposite of LEFT JOIN. It returns all records from the right table (Orders) and matching records from the left table (Customers).
Example Query: Get All Orders and Customer Names
SELECT Customers.Name, Orders.Product
FROM Customers
RIGHT JOIN Orders ON Customers.CustomerID = Orders.CustomerID;
RIGHT JOIN Result
Name | Product |
---|---|
Alice | Laptop |
Bob | Phone |
NULL | Tablet |
β A NULL value appears because Order 103 has no matching customer.
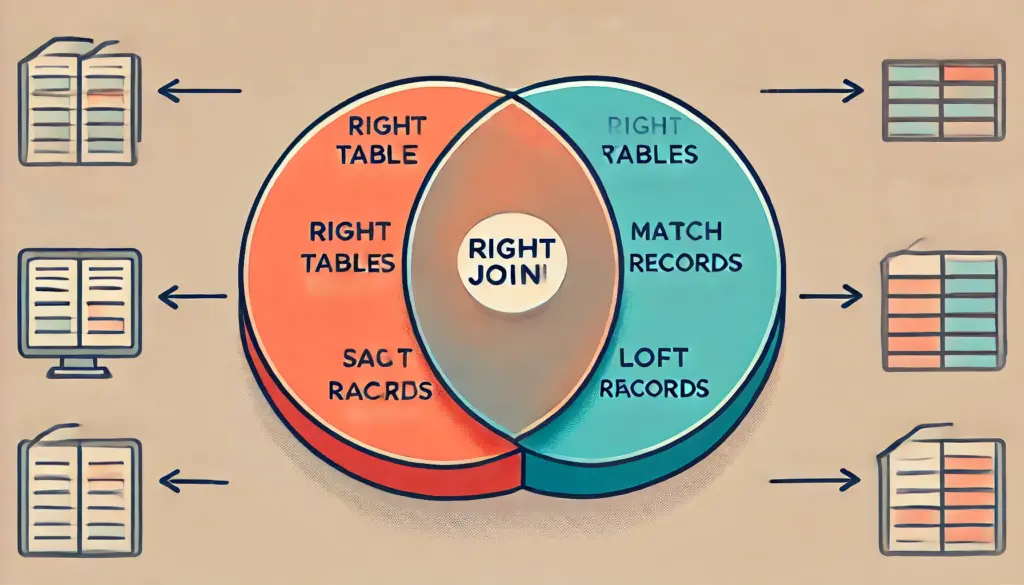
π 5. FULL JOIN β Returning All Records from Both Tables
The FULL JOIN returns all records from both tables, with NULLs for non-matching entries.
Example Query: Get All Customers and All Orders
SELECT Customers.Name, Orders.Product
FROM Customers
FULL JOIN Orders ON Customers.CustomerID = Orders.CustomerID;
FULL JOIN Result
Name | Product |
---|---|
Alice | Laptop |
Bob | Phone |
Charlie | NULL |
NULL | Tablet |
β Includes unmatched records from both tables.
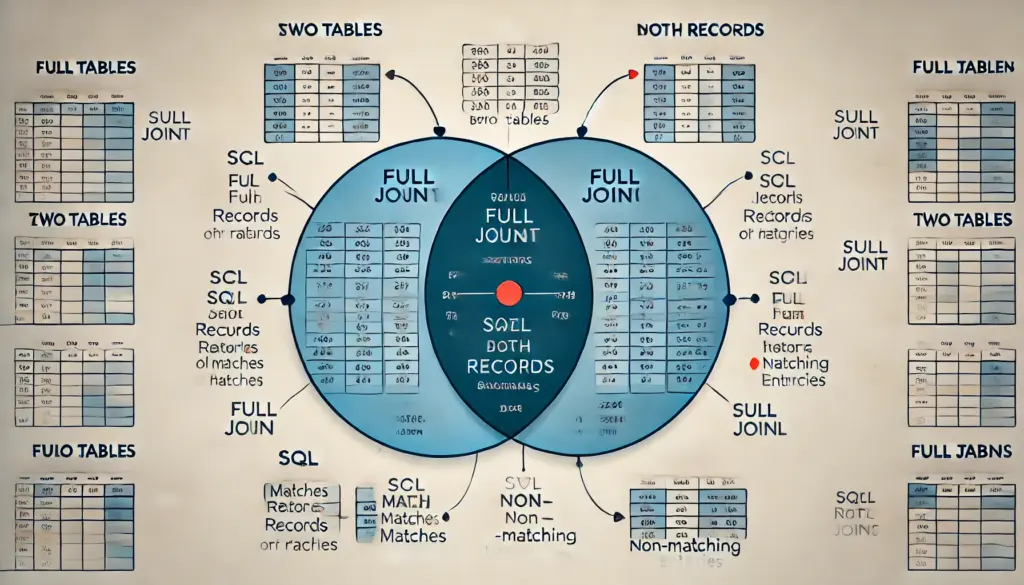
π 6. Choosing the Right SQL Join
JOIN Type | Returns | Best Use Case |
---|---|---|
INNER JOIN | Only matching records | When you only need related data from both tables |
LEFT JOIN | All from the left table + matches | When you want all records from the left table even if no match |
RIGHT JOIN | All from the right table + matches | When you need all data from the right table |
FULL JOIN | All records from both tables | When you want everything, even if no match exists |
π Final Thoughts: Why Master SQL Joins?
SQL Joins are essential for working with relational databases and can help in:
β Data analysis and reporting π
β Merging customer, sales, and product data π’
β Optimizing queries for business insights π
Next up in the series: “SQL Group By & Aggregate Functions: COUNT, SUM, AVG, MIN, MAX Explained” β Stay tuned! π